Are you familiar with the Add-to-Calendar widget that shows up in confirmation emails and web pages after a user registers to an event or a meeting? the widget enables the user to quickly add the event to their favorite calendar, and usually looks something like this:
If you were brave enough to click above on the calendar you're using, you saw an 'add-to-calendar' page show up that's pre-populated with some info. And if you'd like to have the ability to quickly create such widgets for your own audiences, then you're in luck, because today I'll be sharing the code for an online form that you can use to generate these widgets. The output page would look like this:
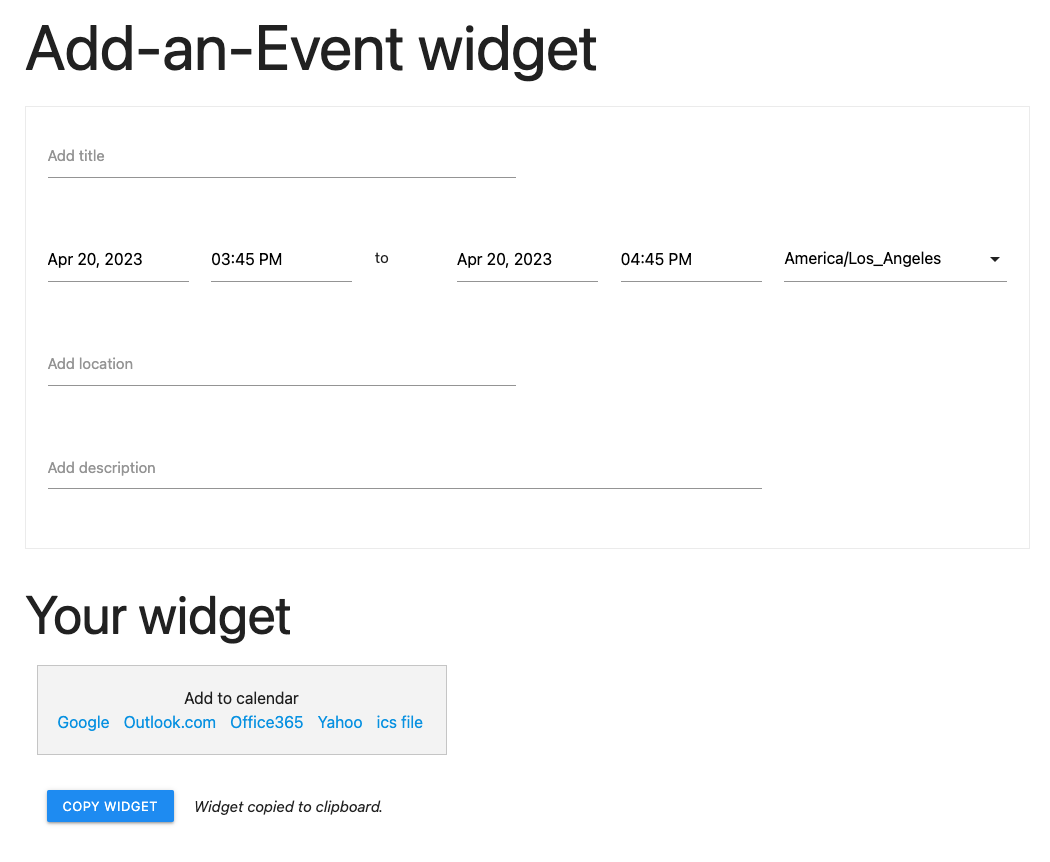
The ICS file
The widget-creation form can be saved in a standard HTML file that you can keep right on your computer's hard-drive. However, if you'd like the widget to also contain a link to download an ICS file (this is a file that enables users to add the event to their offline calendar, like Apple Calendar) then you need a web server that can respond to GET requests and direct the browser to download the file (instead of attempting to open it in the browser.)
The reason you need a web server to download the file is because the standard way of including the ics data in a "data link" doesn't work with numerous email software providers. Gmail, for instance, strips off any href attribute of an anchor link that doesn't point to a server.
And so, because we want to enable the ICS file, I'm going to deploy the form in a Google Apps Script web app. The app will both serve the form and respond to ICS file requests.
The form
Here's the code for the form. Please watch my video at the top of this page if you need help understanding any of the code.
<!DOCTYPE html>
<html>
<head>
<link
href="https://fonts.googleapis.com/icon?family=Material+Icons"
rel="stylesheet"
/>
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css"
/>
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta
name="description"
content="Create a widget to add an event calendar that you can paste in emails or on web pages"
/>
<title>Create "Add Event" widget | Ben Ronkin</title>
</head>
<body>
<main>
<section id="top">
<div class="container">
<h1>Add-an-Event widget</h1>
</div>
</section>
<section id="input">
<div class="container" style="border: 1px solid #eeeeee; padding: 10px">
<form>
<div class="row">
<div class="col s6 input-field">
<input id="title" type="text" />
<label for="title">Add title</label>
</div>
</div>
<div class="row">
<div class="col s2 input-field">
<input
id="fromDate"
name="from-date"
type="text"
class="datepicker cursorPointer"
/>
</div>
<div class="col s2 input-field">
<input
id="fromTime"
name="from-time"
type="text"
class="text"
/>
</div>
<div class="col s1" style="margin-top: 25px">to</div>
<div class="col s2 input-field">
<input
id="toDate"
name="to-date"
type="text"
class="datepicker cursorPointer"
/>
</div>
<div class="col s2 input-field">
<input id="toTime" name="to-time" type="text" class="text" />
</div>
<div class="input-field col s3">
<select id="timezone">
<!-- <option selected disabled>Select timezone</option> -->
</select>
</div>
</div>
<div class="row">
<div class="col s6 input-field">
<input id="location" type="text" />
<label for="location">Add location</label>
</div>
</div>
<div class="row">
<div class="col s9 input-field">
<textarea
id="description"
class="materialize-textarea"
></textarea>
<label for="description">Add description</label>
</div>
</div>
</form>
</div>
</section>
<div class="container">
<h2>Your widget</h2>
</div>
<section id="widget">
<div class="container">
<div class="row">
<div class="col s6">
<div id="widget-container">
<div
style="
border: 1px solid #cccccc;
background-color: #f5f5f5;
padding: 20px;
width: 410px;
font-size: 16px;
"
>
<div style="width: 100%; text-align: center">
Add to calendar
</div>
<div style="width: 100%">
<a style="text-decoration: none; margin-right: 10px" href=""
>Google</a
>
<a style="text-decoration: none; margin-right: 10px" href=""
>Outlook.com</a
>
<a style="text-decoration: none; margin-right: 10px" href=""
>Office365</a
>
<a style="text-decoration: none; margin-right: 10px" href=""
>Yahoo</a
>
<a style="text-decoration: none" href="">ics file</a>
</div>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col s2 input-field" style="margin-left: 10px">
<button class="btn-small blue">Copy Widget</button>
</div>
<div
class="col s8"
style="margin: 20px 0 0 -20px; font-style: italic"
>
<span id="message"
>Widget links update automatically when you edit the
fields.</span
>
</div>
</div>
</div>
</section>
</main>
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
<script>
document.addEventListener('DOMContentLoaded', function () {
// Globals
const title = document.querySelector('#title');
const fromDate = document.querySelector('#fromDate');
const fromTime = document.querySelector('#fromTime');
const toDate = document.querySelector('#toDate');
const toTime = document.querySelector('#toTime');
const timezone = document.querySelector('#timezone');
const location = document.querySelector('#location');
const description = document.querySelector('#description');
const widget = document.querySelector('#widget-container');
const button = document.querySelector('button');
const message = document.querySelector('#message');
let from = new Date();
let to = new Date();
let fromUtc;
let toUtc;
let utcOffsets = {};
function initForm() {
const minutesNow = from.getMinutes();
if (minutesNow <= 15) {
from.setMinutes(15);
} else if (minutesNow <= 30) {
from.setMinutes(30);
} else if (minutesNow <= 45) {
from.setMinutes(45);
} else {
from.setHours(from.getHours() + 1, 0);
}
const today = from.toLocaleDateString('en-US', {
year: 'numeric',
month: 'short',
day: 'numeric',
});
fromDate.value = today;
toDate.value = today;
fromTime.value = from.toLocaleTimeString('en-US', {
hour: '2-digit',
minute: '2-digit',
});
to.setHours(from.getHours() + 1, from.getMinutes());
toTime.value = to.toLocaleTimeString('en-US', {
hour: '2-digit',
minute: '2-digit',
});
}
function pad(x, digits = 2) {
return String(x).padStart(digits, 0);
}
function getUtcString(date) {
const dateWithTimeZoneString =
String(date.getFullYear()) +
'-' +
String(date.getMonth() + 1).padStart(2, 0) +
'-' +
String(date.getDate()).padStart(2, 0) +
'T' +
String(date.getHours()).padStart(2, 0) +
':' +
String(date.getMinutes()).padStart(2, 0) +
':00.000' +
utcOffsets[timezone.value];
const dateWithTimezone = new Date(dateWithTimeZoneString);
let utcString = dateWithTimezone.toISOString();
// Remove milliseconds before returning.
utcString = utcString.split('.')[0] + 'Z';
// Remove dashes and colons
utcString = utcString.replace(/[-:]/g, '');
return utcString;
}
async function setUtcOffset(timezone) {
if (!utcOffsets[timezone]) {
const resp = await fetch(
'https://worldtimeapi.org/api/timezone/' + timezone
);
const jsn = await resp.json();
if (jsn && jsn.utc_offset) {
utcOffsets[timezone] = jsn.utc_offset;
}
}
console.log(utcOffsets);
}
function updateLinks() {
from = new Date(fromDate.value);
let [hh, mm] = fromTime.value.split(/[^\d]/).map((e) => parseInt(e));
if (fromTime.value.toLowerCase().includes('p') && hh < 12) {
hh += 12;
}
from.setHours(hh, mm);
to = new Date(toDate.value);
[hh, mm] = toTime.value.split(/[^\d]/).map((e) => parseInt(e));
if (toTime.value.toLowerCase().includes('p') && hh < 12) {
hh += 12;
}
to.setHours(hh, mm);
const anchorEls = widget.querySelectorAll('a');
anchorEls[0].href = genGoogle();
anchorEls[1].href = genOutlook();
anchorEls[2].href = genOffice365();
anchorEls[3].href = genYahoo();
anchorEls[4].href = genIcs();
}
function genGoogle() {
const url =
'https://www.google.com/calendar/render?action=TEMPLATE' +
'&text=' +
title.value.replace(/\s/g, '+') +
'&dates=' +
getUtcString(from) +
'/' +
getUtcString(to) +
'&location=' +
location.value.replace(/\s/g, '+') +
'&details=' +
description.value.replace(/\s/g, '+');
return url;
}
function genOutlook() {
return genMicrosoft('https://outlook.live.com');
}
function genOffice365() {
return genMicrosoft('https://outlook.office.com/');
}
function genMicrosoft(domain) {
const url =
domain +
'/calendar/0/deeplink/compose?path=%2Fcalendar%2Faction%2Fcompose&rru=addevent' +
'&subject=' +
title.value.replace(/\s/g, '%20') +
'&startdt=' +
String(from.getFullYear()) +
'-' +
pad(from.getMonth() + 1) +
'-' +
pad(from.getDate()) +
'T' +
pad(from.getHours()) +
'%3A' +
pad(from.getMinutes()) +
'%3A' +
'00' +
utcOffsets[timezone.value] +
'&enddt=' +
String(to.getFullYear()) +
'-' +
pad(to.getMonth() + 1) +
'-' +
pad(to.getDate()) +
'T' +
pad(to.getHours()) +
'%3A' +
pad(to.getMinutes()) +
'%3A' +
'00' +
utcOffsets[timezone.value] +
'&location=' +
location.value.replace(/\s/g, '%20') +
'&body=' +
description.value.replace(/\s/g, '%20');
return url;
}
function genYahoo() {
const url =
'https://calendar.yahoo.com/?v=60' +
'&title=' +
title.value.replace(/\s/g, '%20') +
'&st=' +
getUtcString(from) +
'&et=' +
getUtcString(to) +
'&in_loc=' +
location.value.replace(/\s/g, '%20') +
'&desc=' +
description.value.replace(/\s/g, '%20');
return url;
}
function genIcs() {
const now = new Date();
const ics =
'BEGIN:VCALENDAR\n' +
'PRODID:-//benronkin.com//EN\n' +
'VERSION:2.0\n' +
'CALSCALE:GREGORIAN\n' +
'METHOD:PUBLISH\n' +
'BEGIN:VEVENT\n' +
'DTSTAMP:' +
getUtcString(now) +
'\n' +
'DTSTART:' +
getUtcString(from) +
'\n' +
'DTEND:' +
getUtcString(to) +
'\n' +
'UID:' +
now.getTime() +
'@benronkin.com\n' +
'SUMMARY:' +
title.value +
'\n' +
'DESCRIPTION:' +
description.value +
'\n' +
'LOCATION:' +
location.value +
'\n' +
'END:VEVENT\n' +
'END:VCALENDAR\n';
const icsUrl =
'paste-here-the-URL-of-your-google-apps-script-web-app' +
'?ics=' +
encodeURIComponent(ics);
return icsUrl;
// return 'data:text/calendar;charset=utf8,' + encodeURIComponent(ics);
}
function copyWidget() {
navigator.clipboard.writeText(widget.innerHTML);
message.innerText = 'Widget copied to clipboard.';
}
function populateTimeZone() {
const tzs = [
'Africa/Abidjan',
'Africa/Accra',
'Africa/Addis_Ababa',
'Africa/Algiers',
'Africa/Asmara',
'Africa/Asmera',
'Africa/Bamako',
'Africa/Bangui',
'Africa/Banjul',
'Africa/Bissau',
'Africa/Blantyre',
'Africa/Brazzaville',
'Africa/Bujumbura',
'Africa/Cairo',
'Africa/Casablanca',
'Africa/Ceuta',
'Africa/Conakry',
'Africa/Dakar',
'Africa/Dar_es_Salaam',
'Africa/Djibouti',
'Africa/Douala',
'Africa/El_Aaiun',
'Africa/Freetown',
'Africa/Gaborone',
'Africa/Harare',
'Africa/Johannesburg',
'Africa/Juba',
'Africa/Kampala',
'Africa/Khartoum',
'Africa/Kigali',
'Africa/Kinshasa',
'Africa/Lagos',
'Africa/Libreville',
'Africa/Lome',
'Africa/Luanda',
'Africa/Lubumbashi',
'Africa/Lusaka',
'Africa/Malabo',
'Africa/Maputo',
'Africa/Maseru',
'Africa/Mbabane',
'Africa/Mogadishu',
'Africa/Monrovia',
'Africa/Nairobi',
'Africa/Ndjamena',
'Africa/Niamey',
'Africa/Nouakchott',
'Africa/Ouagadougou',
'Africa/Porto-Novo',
'Africa/Sao_Tome',
'Africa/Timbuktu',
'Africa/Tripoli',
'Africa/Tunis',
'Africa/Windhoek',
'America/Adak',
'America/Anchorage',
'America/Anguilla',
'America/Antigua',
'America/Araguaina',
'America/Argentina/Buenos_Aires',
'America/Argentina/Catamarca',
'America/Argentina/ComodRivadavia',
'America/Argentina/Cordoba',
'America/Argentina/Jujuy',
'America/Argentina/La_Rioja',
'America/Argentina/Mendoza',
'America/Argentina/Rio_Gallegos',
'America/Argentina/Salta',
'America/Argentina/San_Juan',
'America/Argentina/San_Luis',
'America/Argentina/Tucuman',
'America/Argentina/Ushuaia',
'America/Aruba',
'America/Asuncion',
'America/Atikokan',
'America/Atka',
'America/Bahia',
'America/Bahia_Banderas',
'America/Barbados',
'America/Belem',
'America/Belize',
'America/Blanc-Sablon',
'America/Boa_Vista',
'America/Bogota',
'America/Boise',
'America/Buenos_Aires',
'America/Cambridge_Bay',
'America/Campo_Grande',
'America/Cancun',
'America/Caracas',
'America/Catamarca',
'America/Cayenne',
'America/Cayman',
'America/Chicago',
'America/Chihuahua',
'America/Ciudad_Juarez',
'America/Coral_Harbour',
'America/Cordoba',
'America/Costa_Rica',
'America/Creston',
'America/Cuiaba',
'America/Curacao',
'America/Danmarkshavn',
'America/Dawson',
'America/Dawson_Creek',
'America/Denver',
'America/Detroit',
'America/Dominica',
'America/Edmonton',
'America/Eirunepe',
'America/El_Salvador',
'America/Ensenada',
'America/Fort_Nelson',
'America/Fort_Wayne',
'America/Fortaleza',
'America/Glace_Bay',
'America/Godthab',
'America/Goose_Bay',
'America/Grand_Turk',
'America/Grenada',
'America/Guadeloupe',
'America/Guatemala',
'America/Guayaquil',
'America/Guyana',
'America/Halifax',
'America/Havana',
'America/Hermosillo',
'America/Indiana/Indianapolis',
'America/Indiana/Knox',
'America/Indiana/Marengo',
'America/Indiana/Petersburg',
'America/Indiana/Tell_City',
'America/Indiana/Vevay',
'America/Indiana/Vincennes',
'America/Indiana/Winamac',
'America/Indianapolis',
'America/Inuvik',
'America/Iqaluit',
'America/Jamaica',
'America/Jujuy',
'America/Juneau',
'America/Kentucky/Louisville',
'America/Kentucky/Monticello',
'America/Knox_IN',
'America/Kralendijk',
'America/La_Paz',
'America/Lima',
'America/Los_Angeles',
'America/Louisville',
'America/Lower_Princes',
'America/Maceio',
'America/Managua',
'America/Manaus',
'America/Marigot',
'America/Martinique',
'America/Matamoros',
'America/Mazatlan',
'America/Mendoza',
'America/Menominee',
'America/Merida',
'America/Metlakatla',
'America/Mexico_City',
'America/Miquelon',
'America/Moncton',
'America/Monterrey',
'America/Montevideo',
'America/Montreal',
'America/Montserrat',
'America/Nassau',
'America/New_York',
'America/Nipigon',
'America/Nome',
'America/Noronha',
'America/North_Dakota/Beulah',
'America/North_Dakota/Center',
'America/North_Dakota/New_Salem',
'America/Nuuk',
'America/Ojinaga',
'America/Panama',
'America/Pangnirtung',
'America/Paramaribo',
'America/Phoenix',
'America/Port_of_Spain',
'America/Port-au-Prince',
'America/Porto_Acre',
'America/Porto_Velho',
'America/Puerto_Rico',
'America/Punta_Arenas',
'America/Rainy_River',
'America/Rankin_Inlet',
'America/Recife',
'America/Regina',
'America/Resolute',
'America/Rio_Branco',
'America/Rosario',
'America/Santa_Isabel',
'America/Santarem',
'America/Santiago',
'America/Santo_Domingo',
'America/Sao_Paulo',
'America/Scoresbysund',
'America/Shiprock',
'America/Sitka',
'America/St_Barthelemy',
'America/St_Johns',
'America/St_Kitts',
'America/St_Lucia',
'America/St_Thomas',
'America/St_Vincent',
'America/Swift_Current',
'America/Tegucigalpa',
'America/Thule',
'America/Thunder_Bay',
'America/Tijuana',
'America/Toronto',
'America/Tortola',
'America/Vancouver',
'America/Virgin',
'America/Whitehorse',
'America/Winnipeg',
'America/Yakutat',
'America/Yellowknife',
'Antarctica/Casey',
'Antarctica/Davis',
'Antarctica/DumontDUrville',
'Antarctica/Macquarie',
'Antarctica/Mawson',
'Antarctica/McMurdo',
'Antarctica/Palmer',
'Antarctica/Rothera',
'Antarctica/South_Pole',
'Antarctica/Syowa',
'Antarctica/Troll',
'Antarctica/Vostok',
'Arctic/Longyearbyen',
'Asia/Aden',
'Asia/Almaty',
'Asia/Amman',
'Asia/Anadyr',
'Asia/Aqtau',
'Asia/Aqtobe',
'Asia/Ashgabat',
'Asia/Ashkhabad',
'Asia/Atyrau',
'Asia/Baghdad',
'Asia/Bahrain',
'Asia/Baku',
'Asia/Bangkok',
'Asia/Barnaul',
'Asia/Beirut',
'Asia/Bishkek',
'Asia/Brunei',
'Asia/Calcutta',
'Asia/Chita',
'Asia/Choibalsan',
'Asia/Chongqing',
'Asia/Chungking',
'Asia/Colombo',
'Asia/Dacca',
'Asia/Damascus',
'Asia/Dhaka',
'Asia/Dili',
'Asia/Dubai',
'Asia/Dushanbe',
'Asia/Famagusta',
'Asia/Gaza',
'Asia/Harbin',
'Asia/Hebron',
'Asia/Ho_Chi_Minh',
'Asia/Hong_Kong',
'Asia/Hovd',
'Asia/Irkutsk',
'Asia/Istanbul',
'Asia/Jakarta',
'Asia/Jayapura',
'Asia/Jerusalem',
'Asia/Kabul',
'Asia/Kamchatka',
'Asia/Karachi',
'Asia/Kashgar',
'Asia/Kathmandu',
'Asia/Katmandu',
'Asia/Khandyga',
'Asia/Kolkata',
'Asia/Krasnoyarsk',
'Asia/Kuala_Lumpur',
'Asia/Kuching',
'Asia/Kuwait',
'Asia/Macao',
'Asia/Macau',
'Asia/Magadan',
'Asia/Makassar',
'Asia/Manila',
'Asia/Muscat',
'Asia/Nicosia',
'Asia/Novokuznetsk',
'Asia/Novosibirsk',
'Asia/Omsk',
'Asia/Oral',
'Asia/Phnom_Penh',
'Asia/Pontianak',
'Asia/Pyongyang',
'Asia/Qatar',
'Asia/Qostanay',
'Asia/Qyzylorda',
'Asia/Rangoon',
'Asia/Riyadh',
'Asia/Saigon',
'Asia/Sakhalin',
'Asia/Samarkand',
'Asia/Seoul',
'Asia/Shanghai',
'Asia/Singapore',
'Asia/Srednekolymsk',
'Asia/Taipei',
'Asia/Tashkent',
'Asia/Tbilisi',
'Asia/Tehran',
'Asia/Tel_Aviv',
'Asia/Thimbu',
'Asia/Thimphu',
'Asia/Tokyo',
'Asia/Tomsk',
'Asia/Ujung_Pandang',
'Asia/Ulaanbaatar',
'Asia/Ulan_Bator',
'Asia/Urumqi',
'Asia/Ust-Nera',
'Asia/Vientiane',
'Asia/Vladivostok',
'Asia/Yakutsk',
'Asia/Yangon',
'Asia/Yekaterinburg',
'Asia/Yerevan',
'Atlantic/Azores',
'Atlantic/Bermuda',
'Atlantic/Canary',
'Atlantic/Cape_Verde',
'Atlantic/Faeroe',
'Atlantic/Faroe',
'Atlantic/Jan_Mayen',
'Atlantic/Madeira',
'Atlantic/Reykjavik',
'Atlantic/South_Georgia',
'Atlantic/St_Helena',
'Atlantic/Stanley',
'Australia/ACT',
'Australia/Adelaide',
'Australia/Brisbane',
'Australia/Broken_Hill',
'Australia/Canberra',
'Australia/Currie',
'Australia/Darwin',
'Australia/Eucla',
'Australia/Hobart',
'Australia/LHI',
'Australia/Lindeman',
'Australia/Lord_Howe',
'Australia/Melbourne',
'Australia/North',
'Australia/NSW',
'Australia/Perth',
'Australia/Queensland',
'Australia/South',
'Australia/Sydney',
'Australia/Tasmania',
'Australia/Victoria',
'Australia/West',
'Australia/Yancowinna',
'Brazil/Acre',
'Brazil/DeNoronha',
'Brazil/East',
'Brazil/West',
'Canada/Atlantic',
'Canada/Central',
'Canada/Eastern',
'Canada/Mountain',
'Canada/Newfoundland',
'Canada/Pacific',
'Canada/Saskatchewan',
'Canada/Yukon',
'Chile/Continental',
'Chile/EasterIsland',
'Cuba',
'Egypt',
'Eire',
'Europe/Amsterdam',
'Europe/Andorra',
'Europe/Astrakhan',
'Europe/Athens',
'Europe/Belfast',
'Europe/Belgrade',
'Europe/Berlin',
'Europe/Bratislava',
'Europe/Brussels',
'Europe/Bucharest',
'Europe/Budapest',
'Europe/Busingen',
'Europe/Chisinau',
'Europe/Copenhagen',
'Europe/Dublin',
'Europe/Gibraltar',
'Europe/Guernsey',
'Europe/Helsinki',
'Europe/Isle_of_Man',
'Europe/Istanbul',
'Europe/Jersey',
'Europe/Kaliningrad',
'Europe/Kiev',
'Europe/Kirov',
'Europe/Kyiv',
'Europe/Lisbon',
'Europe/Ljubljana',
'Europe/London',
'Europe/Luxembourg',
'Europe/Madrid',
'Europe/Malta',
'Europe/Mariehamn',
'Europe/Minsk',
'Europe/Monaco',
'Europe/Moscow',
'Europe/Nicosia',
'Europe/Oslo',
'Europe/Paris',
'Europe/Podgorica',
'Europe/Prague',
'Europe/Riga',
'Europe/Rome',
'Europe/Samara',
'Europe/San_Marino',
'Europe/Sarajevo',
'Europe/Saratov',
'Europe/Simferopol',
'Europe/Skopje',
'Europe/Sofia',
'Europe/Stockholm',
'Europe/Tallinn',
'Europe/Tirane',
'Europe/Tiraspol',
'Europe/Ulyanovsk',
'Europe/Uzhgorod',
'Europe/Vaduz',
'Europe/Vatican',
'Europe/Vienna',
'Europe/Vilnius',
'Europe/Volgograd',
'Europe/Warsaw',
'Europe/Zagreb',
'Europe/Zaporozhye',
'Europe/Zurich',
'GB',
'GB-Eire',
'Hongkong',
'Iceland',
'Indian/Antananarivo',
'Indian/Chagos',
'Indian/Christmas',
'Indian/Cocos',
'Indian/Comoro',
'Indian/Kerguelen',
'Indian/Mahe',
'Indian/Maldives',
'Indian/Mauritius',
'Indian/Mayotte',
'Indian/Reunion',
'Iran',
'Israel',
'Jamaica',
'Japan',
'Kwajalein',
'Libya',
'Mexico/BajaNorte',
'Mexico/BajaSur',
'Mexico/General',
'Navajo',
'NZ',
'NZ-CHAT',
'Pacific/Apia',
'Pacific/Auckland',
'Pacific/Bougainville',
'Pacific/Chatham',
'Pacific/Chuuk',
'Pacific/Easter',
'Pacific/Efate',
'Pacific/Enderbury',
'Pacific/Fakaofo',
'Pacific/Fiji',
'Pacific/Funafuti',
'Pacific/Galapagos',
'Pacific/Gambier',
'Pacific/Guadalcanal',
'Pacific/Guam',
'Pacific/Honolulu',
'Pacific/Johnston',
'Pacific/Kanton',
'Pacific/Kiritimati',
'Pacific/Kosrae',
'Pacific/Kwajalein',
'Pacific/Majuro',
'Pacific/Marquesas',
'Pacific/Midway',
'Pacific/Nauru',
'Pacific/Niue',
'Pacific/Norfolk',
'Pacific/Noumea',
'Pacific/Pago_Pago',
'Pacific/Palau',
'Pacific/Pitcairn',
'Pacific/Pohnpei',
'Pacific/Ponape',
'Pacific/Port_Moresby',
'Pacific/Rarotonga',
'Pacific/Saipan',
'Pacific/Samoa',
'Pacific/Tahiti',
'Pacific/Tarawa',
'Pacific/Tongatapu',
'Pacific/Truk',
'Pacific/Wake',
'Pacific/Wallis',
'Pacific/Yap',
'Poland',
'Portugal',
'PRC',
'ROC',
'ROK',
'Singapore',
'Turkey',
'US/Alaska',
'US/Aleutian',
'US/Arizona',
'US/Central',
'US/East-Indiana',
'US/Eastern',
'US/Hawaii',
'US/Indiana-Starke',
'US/Michigan',
'US/Mountain',
'US/Pacific',
'US/Samoa',
];
tzs.forEach((tz) => {
const option = document.createElement('option');
option.value = tz;
option.innerText = tz;
timezone.appendChild(option);
});
const userTz = Intl.DateTimeFormat().resolvedOptions().timeZone;
const userOption = document.querySelector(
`option[value="${userTz}"]`
);
userOption.setAttribute('selected', 'selected');
}
async function init() {
populateTimeZone();
await setUtcOffset(timezone.value);
let els = document.querySelectorAll('.datepicker');
M.Datepicker.init(els, {});
els = document.querySelectorAll('select'); // required here
M.FormSelect.init(els, {});
title.addEventListener('change', updateLinks);
fromDate.addEventListener('change', updateLinks);
fromTime.addEventListener('change', updateLinks);
toDate.addEventListener('change', updateLinks);
toTime.addEventListener('change', updateLinks);
location.addEventListener('change', updateLinks);
description.addEventListener('change', updateLinks);
timezone.addEventListener('change', async function (e) {
await setUtcOffset(e.target.value);
updateLinks();
});
button.addEventListener('click', copyWidget);
initForm();
updateLinks();
}
init();
}); // End of DOMContentLoaded
</script>
</body>
</html>
The web app
I recommend that you create a new Google Apps Script file. In it, create an HTML file, and name it "index.html". Then, in "Code.gs", enter the following code:
function doGet(e) {
const icsStr = e.parameter && e.parameter.ics ? e.parameter.ics : null;
if (icsStr) {
const file = DriveApp.createFile(icsStr, 'event.ics');
file.setTrashed(true);
return ContentService.createTextOutput() // Create textOutput Object
.append(file) // Append the text from our csv file
.downloadAsFile('event.ics'); // Have browser download, rather than display
} else {
const htmlTemplate = HtmlService.createTemplateFromFile('index');
const htmlOutput = htmlTemplate.evaluate();
return htmlOutput;
}
}
Again, watch the video to understand how to deploy the script as a web app. Once you deploy it, you want to copy the generated web app URL, go back to your index.html file and paste the URL instead of the 'paste-here-the-URL-of-your-google-apps-script-web-app' string. Then you want to redeploy the web app by selecting a New Version, and you're done.
Once your web app is deployed, you can enter the web app URL in your browser's address bar. You'll be able to change the widget URLs automatically by entering data in the form. Note that once you copy the widget, you can paste it as-is in email messages, but if you add it to HTML pages, you may need to replace the "&" entries in the URLs with "&".